Yesterday, I was playing around with my VS Code settings, which is something normal that I do for fun.
I came across settings for Inlay Type Hints, which is a new feature to me, but apparently has been available since July.
Here’s a quick demo:
You can see that Pylance (VS Code’s Python language server) now displays inferred types for function returns and variables that have not been explicitly type annotated.
The user experience is good but I have a few ideas for improvements:
- It would be great if type inlays had color/mouse-over information before being inserted,
- and if types were also automatically imported when inserted.
For now, I have function return annotation enabled all the time, and turn variable annotation on and off depending on what I’m doing – it can be a bit spammy in dense code, but it’s invaluable for debugging. (It would also be nice to be able to toggle annotations with a click!)
Overall, I think this a really cool feature. It underlines the big improvements in Python tooling – by VS Code in particular – that have been made possible by the gradual introduction of type annotation support to the language.
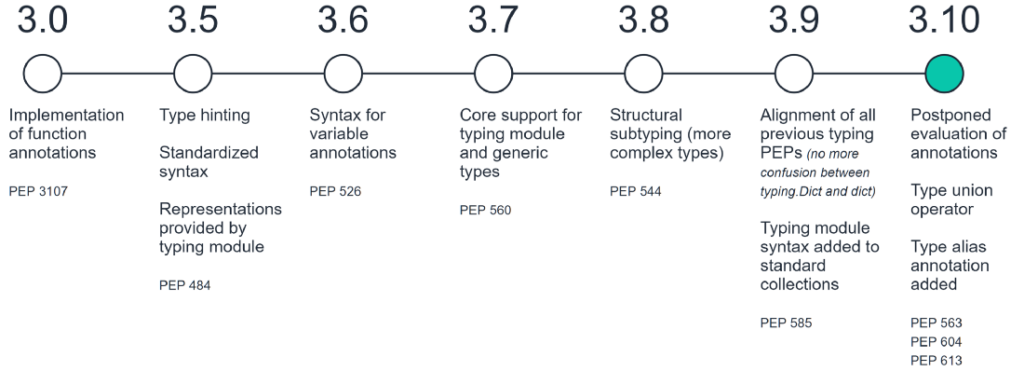
Source: Towards Data Science